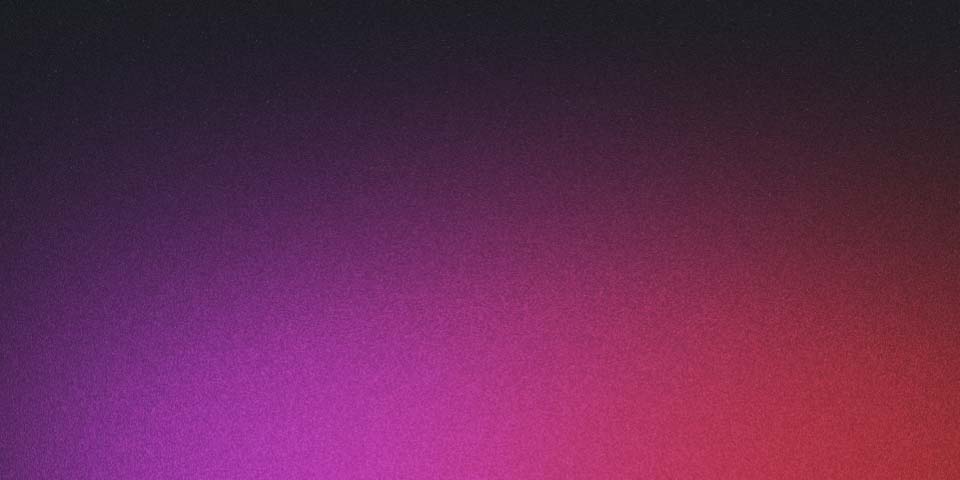
Setting up Gatsby
So my site has been well neglected for the last few years and I thought it was time to bring it back from the dead with new content using a newer platform / static site generator. I’ve been wanting to try Gatsby for some time so what better way than to document setting up the site itself.
Gatsby install
So firstly you’ll need to install the Gatsby CLI globally
npm install -g gatsby-cli
Next create your site and change into the new directory
gatsby new your-site
cd your-site
You’ll notice a fair few files and directories at the root and source. Have a look around they’re not that complicated and you can get familiar with them pretty quickly by looking at the docs (especially the recipes).
Start up your local development server or create a prod build
It’s super easy to get your local dev server running to check out your site.
gatsby develop
then go to localhost:8000 by default (it also starts up a graphql endpoint which you can get more familiar with later). It’s also hot reload so your changes will come through as soon as you save them.
To create a prod build and then run it locally perform the following commands.
gatsby build
gatsby serve
Creating and adding pages from Markdown to your site
I became accustomed to creating my pages in markdown after using Hexo in the past (another static site generator) so I wanted the same easy experience for creating content. There’s a recipe for this on the docs site which is quite thorough although I’ve summarised some of the steps here.
Install the plugins, gatsby-source-filesystem (to read local files) and gatsby-transformer-remark (to parse your markdown).
npm install --save gatsby-source-filesystem
npm install --save gatsby-transformer-remark
I had some trouble initially with npm complaining (npm ERR! Maximum call stack size exceeded
) several times. In the end I deleted my node_modules folder (rm -r node_module
) and then ran the previous commands followed by npm install
again.
Alter your gatsby-config.js file as below (add it in with the other plugins taking car with your commas).
plugins: [
`gatsby-transformer-remark`,
{
resolve: `gatsby-source-filesystem`,
options: {
name: `content`,
path: `${__dirname}/src/content`,
},
},
];
That path is where you’ll keep your markdown-pages.
Add a Markdown post in the src/content
folder as below.
---
title: First Post
date: 2019-11-01
path: /first-post
---
Write up your markdown like you normally would here
Open up the gatsby-node.js
file and put the following in.
const path = require(`path`);
exports.createPages = async ({ actions, graphql }) => {
const { createPage } = actions;
const result = await graphql(`
{
allMarkdownRemark {
edges {
node {
frontmatter {
path
}
}
}
}
}
`);
if (result.errors) {
console.error(result.errors);
}
result.data.allMarkdownRemark.edges.forEach(({ node }) => {
createPage({
path: node.frontmatter.path,
component: path.resolve(`src/templates/post.js`),
});
});
};
You’ll need a template as mentioned in the last line of code. Create this file here src/templates/post.js
and put in the following.
import React from "react";
import { graphql } from "gatsby";
import Layout from "../components/layout";
export default function Template({ data }) {
const { markdownRemark } = data; // data.markdownRemark holds your post data
const { frontmatter, html } = markdownRemark;
return (
<Layout>
<div className="blog-post">
<h1>{frontmatter.title}</h1>
<h2>{frontmatter.date}</h2>
<div
className="blog-post-content"
dangerouslySetInnerHTML={{ __html: html }}
/>
</div>
</Layout>
);
}
export const pageQuery = graphql`
query ($path: String!) {
markdownRemark(frontmatter: { path: { eq: $path } }) {
html
frontmatter {
date(formatString: "MMMM DD, YYYY")
path
title
}
}
}
`;
Get the dev server running again and we should see our Markdown content wrapped in our layout by visiting the path of the post e.g. http://localhost:8000/first-post
. If you had it running already you may need to stop and start it again to pick up the page.
So this is the basic site up and running with Markdown although obviously with all the standard layout and styles. In the next posts I’ll look into looping through the files to display the latest posts on the homepage.